
Packet Templates
Create Complex Binary Packets Interactively in a Property GridCreating binary packets for transmission can be challenging for two key reasons:
-
Encoding Complexity: Each packet field must be meticulously encoded, ensuring the correct byte order, lengths, and offsets are respected.
-
Checksum Calculations: Many fields, such as checksums, depend on the rest of the packet's content. These fields must be updated every time the packet changes, which makes manual updates both tedious and error-prone.
IO Ninja provides an elegant solution to these challenges. With Packet Templates, you can interactively prepare binary packets in a property grid, and calculated fields (like checksums) are updated automatically, saving time and effort.
Preparing & Sending Modbus Frames
How Does It Work?
First, you describe the packet structure in the Jancy language.
Jancy is highly compatible with C at both the source and ABI levels.
It supports structure packing, bitfields, and even big-endian integers (integers with reversed byte order).
With Jancy, you can describe any binary packet structure just like you would in C/C++ — and even better!
A packet declaration can include methods that perform specific actions on the packet data.
For example:
- Define a method like
updateChecksum()
to automatically recalculate checksum fields. - Create a method like
setTargetAddress(string_t addressString)
to encode an address from user input.
Actions can be marked with [autorun]
, ensuring they execute automatically whenever the packet changes.
Once the packet structure is defined, IO Ninja parses it and generates an interactive property grid.
- Modify packet fields directly with intuitive editors or dropdown menus.
- No need to manually calculate offsets, lengths, or byte order — everything is handled automatically.
- Fields like checksums and payload lengths update in real-time.
- Additional utility actions can be triggered with a simple mouse click.
Example
Consider the following packet declaration:
import "crc16.jnc"
[
packetTemplate,
displayName = "My packet"
]
struct MyPacket {
[ displayName = "32-bit big-endian" ]
bigendian int32_t m_id;
[ displayName = "Name" ]
char m_name[8];
[ displayName = "Length" ]
uint16_t m_length;
[ displayName = "CRC-16" ]
uint16_t m_checksum;
[
userAction = "Update length & checksum",
autorun = "Auto-update"
]
void update() {
m_length = dynamic sizeof(this);
m_checksum = 0;
m_checksum = crc16_ansi(this, m_length);
}
}
The declaration above generates the following packet template:
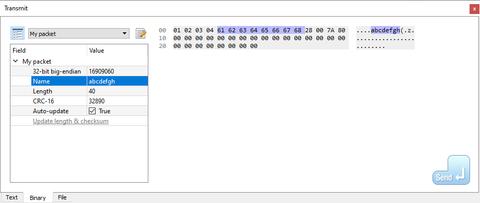
Notice the userAction
and autorun
attributes for the update()
method.
These attributes create a clickable hyperlink that runs automatically whenever the packet changes.
You can temporarily disable Auto-update
by unchecking the corresponding checkbox — useful for testing packets with intentionally broken checksums.
Stock Libraries
IO Ninja includes several stock packet libraries for common protocols:
Library | Packets | ||||||
---|---|---|---|---|---|---|---|
TCP/IP |
| ||||||
Modbus RTU |
| ||||||
Modbus TCP |
|
For example, if you need to send custom raw UDP packets using Pcap or read Modbus registers from a slave device over an RS-485 bus — IO Ninja has you covered.
Alternatively, use the stock library sources as a reference to define custom packet structures for your protocol.
(Stock packet templates are located in $IONINJA_ROOT_DIR/scripts/packets/
.)